jQuery CSS Class playground
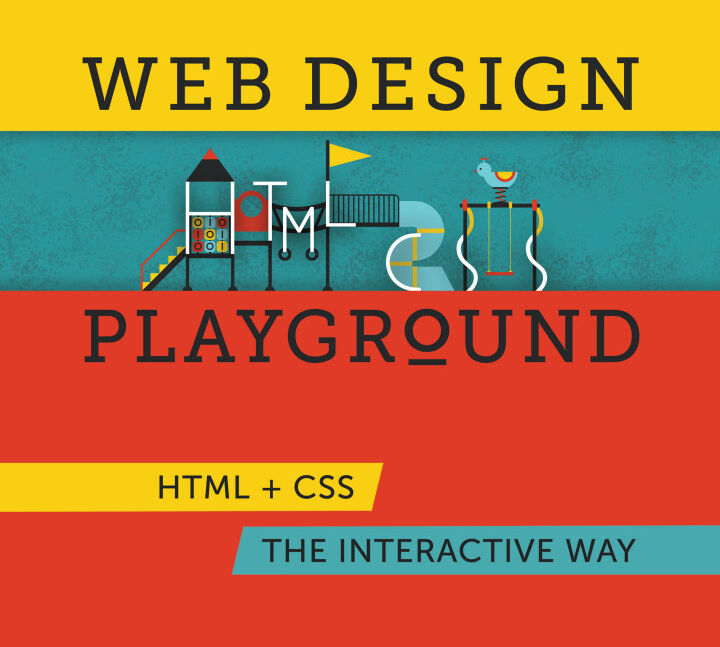
Today we will play with jQuery CSS class methods such as addClass, removeClass, hasClass, and toggleClass and learn some basic implementation to modify the HTML styles on the fly.
jQuery CSS Class Methods:
Let’s understand this is a small piece of code. First things first, You must include the jQuery library in the <head>…</head> tag.
<script src=PATH_TO_JQUERY/jquery.min.js></script>
Now our page has the jQuery library so let’s add some HTML and CSS to play with the jQuery CSS Class methods.
HTML Code:
<div id="elem">
This is a content panel
</div>
<p>
<a href="#" >addClass</a> /
<a href="#" >removeClass</a> /
<a href="#" >toggleClass</a> /
<a href="#" >hasClass</a>
</p>
Here we have a DIV tag with ID set to element and we will use this ID to play with jQuery CSS class methods. Below that, we have a paragraph element and four links with different class names. Let’s write some CSS code and I will explain the functionality of each link when we add jQuery code.
CSS Code
#elem{ width: 400px; text-align: center; padding: 20px 0; background: #ffffff; border: 1px solid #ddd; margin: 100px auto 30px auto; } .hover{ background: #cc0000 !important; color: #fff !important; border: 1px solid #990000 !important; }
In the CSS styles, I’ve added some styles to our DIV#elem element and also created a class named hover which will change the background, color, and border of the DIV#elem.
Now let’s add some jQuery code and learn how these jQuery CSS methods work.
$(document).ready(function(){ // Add the class $('a.add-class').click(function(){ $("#elem").addClass('hover'); $("#elem").html('Class added ".hover"'); return false; }); // Remove the class $('a.remove-class').click(function(){ $("#elem").removeClass('hover'); $("#elem").html('Class removed ".hover"'); return false; }); // Toggle the class and check if the class has been already added or not $('a.toggle-class').click(function(){ $("#elem").toggleClass('hover'); if($("#elem").hasClass('hover')){ $("#elem").html('Class added ".hover"'); }else{ $("#elem").html('Class removed ".hover"'); } return false; }); // Checks if the class has been already added or not $('a.has-class').click(function(){ if($("#elem").hasClass('hover')){ $("#elem").html('Class found ".hover"'); }else{ $("#elem").html('Class not found ".hover"'); } return false; }); });
In the JQuery code, I’ve used the click trigger for all four links we added in the HTML code above. Also, I’ve used return false; so when we click on the link it should not redirect the user to any page or use # in the current page URL. Also, I’ve added another line of code to modify the HTML of the #elem element when we click on these links. Don’t get confused with the .html() method, this is not required, I just added it to explain the functionality.
The first link with class .add-class will add the .hover class to the #elem element. If you want to add multiple classes with one trigger you can specify different class names separated with a space as we normally do in normal HTML code. Once we click on this link, .hover class will be added to the #elem HTML element and the inner HTML will be changed and show us the result. You can click on the address link above and see it in action.
The second link with class .remove-class will remove the .hover class from the #elem element. If the element doesn’t have a .hover class, it won’t do anything so the remove class will be used only to remove any applied class.
The third link with class .toggleClass will toggle the .hove class on #elem element. If the element does not have a .hover class it will add the same otherwise vice versa. In this code, I’ve used the jQuery CSS method hasClass to check if the element has the .hover class or not. and the content of the #elem element is changed accordingly.
The fourth link will only check if the class .hover is applied to the #elem element or not and change the HTML code of the #elem element accordingly.
I hope this will clarify any doubts on using these methods, in case you have any questions or suggestions, please use the comments below to start a conversation on this topic.
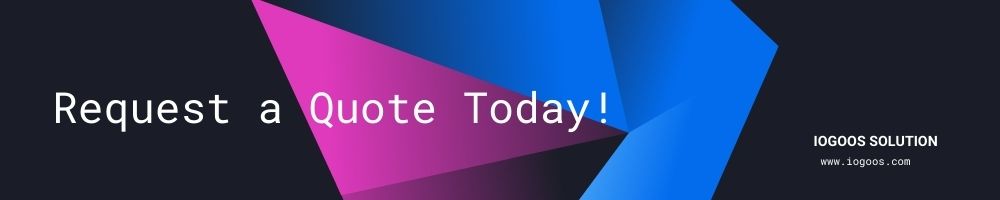
Leave a Reply